Understanding Breadth First Search in Rust: A Complete Guide.
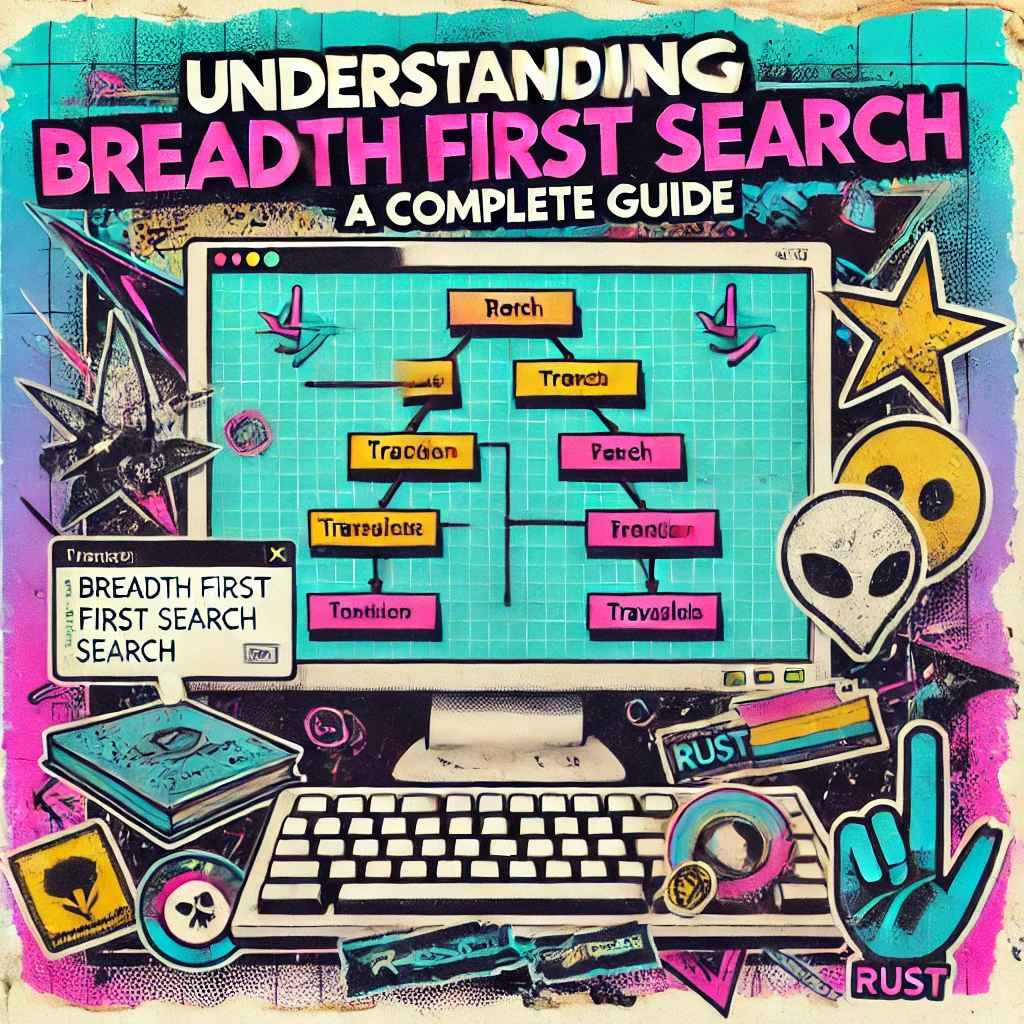
Introduction Breadth First Search is a fundamental tree traversal algorithm that visits all nodes at the current depth before moving to nodes at the next depth level. In this tutorial, we’ll implement BFS in Rust using a binary tree structure. We’ll break down each component and explore how it works. 1. Setting Up the Tree […]
Handling Errors in RUST using Result
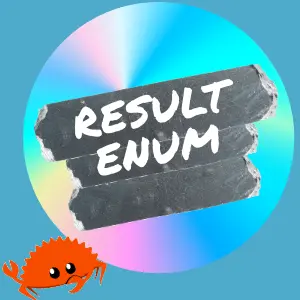
The Result enum in Rust is used to handle operations that may produce an error. It represents the possibility of either success (Ok) or failure (Err) and allows you to handle these outcomes in a controlled way. Result Definition Example enum Result { Ok(T), Err(E), } Here, T represents the type of the success value, […]
How to use Options in RUST

Unlike in other languages where there is a null or None type to represent no data. In Rust we can use Option enum when there is a possibility of having a value or having no value at all arises. It is most commonly used to handle cases where a value might be missing or to […]
ENUMS in RUST Programming
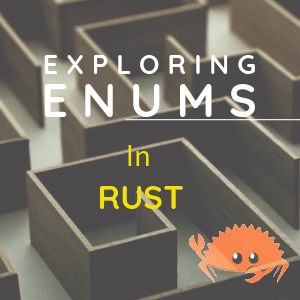
Enum is a symbolic name for a set of values. Enums are treated as data types, and you can use them to create sets of constants for use with variables and properties. It offers an easier way to work with sets of related constants. we use the Keyword enum to define an enum. RUST ENUM […]
Structs in RUST
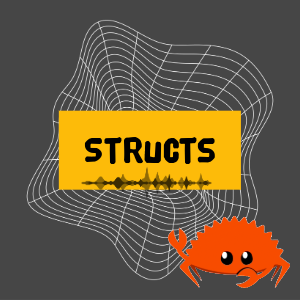
Table of Contents Structs Structs in Rust are similar to structs in other programming languages. They allow you to create custom data types by grouping related data together. Rust has Three types of structs: Named field Struct Tuple Struct Unit Struct RUST struct examples Named Field Struct You can define a struct using the struct […]
Installing RUST in Ubuntu 22.04 LTS
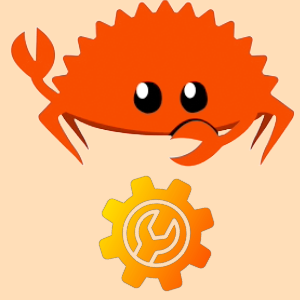
How to install RUST in UBUNTU 22.04 LTS.
RUST Data Type Conversion
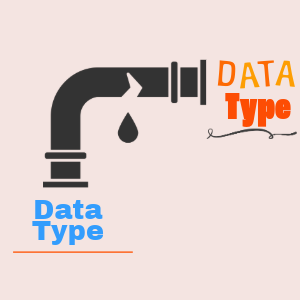
Master Type Conversion in Rust – explicit with ‘as’ and ‘try_into()’, implicit via compiler. Explore small integer promotions, handle Result Types, and navigate key considerations like overflow, precision loss, and Rust’s robust type safety. Elevate your Rust programming proficiency with this concise guide